Data Type's
In Python, a datatype is a classification that specifies which type of value a variable can hold. The use of datatypes in Python is crucial for defining the kind of data a variable can store and for determining the operations that can be performed on it. Here are some key aspects related to datatypes in Python
Definition of Datatype
- A datatype in Python refers to the type or category of data a variable can hold.
- Examples of basic datatypes include integers, floats, strings, and booleans.
Uses of Datatypes in Python
- Variable Declaration: Datatypes help in declaring variables with specific types of data.
- Memory Allocation: Different datatypes require varying amounts of memory, optimizing resource usage.
- Operation Validity: Datatypes determine which operations are valid for a given type of data.
- Function Parameters: Datatypes ensure that functions receive the correct type of input parameters.
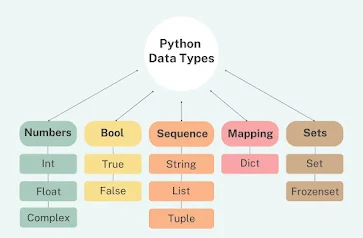
Types of Datatypes in Python
Built-in Datatypes in Python
Python provides several built-in datatypes that cover a wide range of data structures and values. These are ready to use and don't require explicit definition
Numeric Types:
- Integers (
int
) - Floating-point numbers (
float
)
- Complex numbers (
complex
)
- Strings (
str
) - Lists (
list
) - Tuples (
tuple
)
- Sets (
set
)
- Dictionaries (
dict
)
- Booleans (
bool
)
- None (
NoneType
)
Common Datatype Operations:
Type Conversion: Changing the datatype of a variable
Example:
- # Type Conversion num_str = str(num_int)
Indexing and Slicing: Accessing specific elements in sequences. Example: # Indexing and Slicing first_char = string_example[0] sub_list = list_example[1:4]
Advanced Datatype Concepts
Nested Data Structures:
Python allows the creation of complex data structures by nesting different types within one another.
Example:
nested_dict
is a dictionary with nested dictionaries, showcasing the flexibility of Python's datatype system.Custom Datatypes:
Developers can create their own custom datatypes using classes, introducing a higher level of abstraction and encapsulation.
Example:
Point
class defines a custom datatype representing a point in a two-dimensional space. Instances of this class can be created with specific x
and y
coordinates.Dynamic Typing:
Python is dynamically typed, meaning variable types can change during runtime.
Example:
Use Meaningful Variable Names:
Choose variable names that convey the intended datatype and purpose, enhancing code readability.
Example:
num_items
is an integer, and item_names
is a list, making the code more self-explanatory.Type Annotations:
In Python 3.5 and later, type annotations can be used to indicate the expected datatype of a variable.
Example:
add_numbers
takes two integers (a
and b
) as parameters and returns an integer, providing clarity to both developers and tools.User-defined Datatypes in Python
Point
is a custom datatype representing a point in a two-dimensional space. point_instance
is an instance of this datatype with specific x
and y
coordinates.DynamicVariable
class allows the creation of instances with different datatypes, showcasing Python's dynamic typing.Uses of Data types
Data Storage: Data types determine how data is stored in memory. For example, integers are stored differently than floating-point numbers or strings. By specifying the data type of a variable, you can ensure that the variable is stored and manipulated correctly.
Data Validation: Data types are used to validate the input data and ensure that it meets the requirements of the program. For example, if a function expects an integer input, you can check the data type of the input to ensure that it is an integer before proceeding with the operation.
Data Manipulation: Data types define the operations that can be performed on the data. For example, you can perform arithmetic operations on integers and floating-point numbers, but not on strings. Data types determine how these operations are carried out and what the result will be.
Memory Management: Data types are used for memory management, determining how much memory should be allocated for a variable based on its data type. This helps optimize memory usage and improve the performance of the program.
Compatibility: Data types ensure compatibility between different parts of a program. By using consistent data types, you can ensure that data is passed between functions and modules correctly and that operations are performed as expected.
FAQs on Datatypes in Python
Q1: What are datatypes in Python? A1: Datatypes in Python classify the type of data a variable can hold, such as integers, floats, strings, and more.
Q2: How many numeric types does Python have?
A2: Python has three numeric types: integers (int
), floating-point numbers (float
), and complex numbers (complex
).
Q3: What are sequence types in Python?
A3: Sequence types include strings (str
), lists (list
), and tuples (tuple
). They represent ordered collections of items.
Q4: Can you provide an example of set datatype in Python?
A4: Certainly! set_example = {1, 2, 3, 4, 5}
is an example of a set in Python, containing unique, unordered elements.
Q5: Explain the mapping type in Python.
A5: The mapping type is represented by dictionaries (dict
). It consists of key-value pairs, allowing efficient data retrieval.
Q6: What is the boolean datatype used for?
A6: The boolean datatype (bool
) is used for representing truth values, with values True
or False
.
Q7: How does type conversion work in Python?
A7: Type conversion involves changing the datatype of a variable. For example, num_str = str(42)
converts an integer to a string.
Q8: What is dynamic typing in Python? A8: Python is dynamically typed, allowing variables to change types during runtime. For instance, a variable can start as an integer and later become a string.
Q9: How can I create a custom datatype in Python?
A9: You can create a custom datatype by defining a class. For example, a Point
class can represent a point in a two-dimensional space.
Q10: What are the best practices for working with datatypes in Python? A10: Best practices include using meaningful variable names, employing type annotations for clarity, and adhering to conventions for improved readability.
Q11: Can I nest data structures in Python? A11: Yes, Python allows nesting data structures. For example, a dictionary can contain other dictionaries, creating complex, hierarchical structures.
Q12: How can type annotations be used in Python?
A12: Type annotations can be added to function parameters and return values, providing information about the expected datatypes. For instance, def add_numbers(a: int, b: int) -> int
.
Summary
Python offers a rich set of built-in datatypes, including numeric types like integers, floats, and complex numbers. Sequence types like strings, lists, and tuples allow orderly data storage, while sets manage unordered, unique elements. Dictionaries serve as a mapping type for key-value pairs, and booleans and None represent truth values and the absence of a value, respectively.
Point
can represent a point in a two-dimensional space. This flexibility extends to dynamic typing, where instances of user-defined datatypes can change types during runtime.Understanding these concepts and best practices not only empowers developers to work effectively with the broad spectrum of Python datatypes but also facilitates the creation of more readable, maintainable, and expressive code.