What is Python?
Why python ?
Uses Of Python
Web Development: Python's frameworks like Django and Flask are popular for building web applications. They provide tools and libraries for tasks such as URL routing, form processing, and database interaction.
Data Science and Machine Learning: Python is widely used in data science and machine learning due to its simplicity and the availability of libraries like NumPy, pandas, and scikit-learn. These libraries provide tools for data manipulation, analysis, and machine learning model development.
Scripting: Python is often used for scripting tasks, such as automating repetitive tasks, interacting with system resources, and processing text files. Its simple syntax and readability make it well-suited for these tasks.
Scientific Computing: Python is used in scientific computing for tasks such as numerical simulations, data visualization, and data analysis. Libraries like NumPy, SciPy, and matplotlib are commonly used for these purposes.
Game Development: Python is used in game development, particularly for building 2D games. Libraries like Pygame provide tools for developing games with graphics, sound, and user input.
Desktop GUI Applications: Python can be used to create desktop GUI applications using libraries like Tkinter, PyQt, and wxPython. These libraries provide tools for creating windows, buttons, menus, and other GUI elements.
Network Programming: Python's built-in libraries like socket provide tools for network programming, such as creating client-server applications, sending and receiving data over the network, and working with web APIs.
Education: Python is often used in educational settings due to its readability and ease of use. It is used to teach programming concepts to beginners and is the language of choice for introductory programming courses in many universities.
Backend Development: Python is used in backend development for building server-side applications and APIs. Frameworks like Django and Flask are commonly used for this purpose.
DevOps and Automation: Python is used in DevOps for tasks such as configuration management, infrastructure automation, and deployment scripting. Tools like Ansible and SaltStack are written in Python and use Python for their configuration files and scripts.
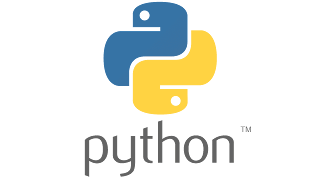
FAQ'S
Basics of Python:
Q: What is Python, and how would you describe it to someone new to programming?
- A: Python is a high-level, interpreted programming language known for its readability and simplicity. It supports multiple programming paradigms and is widely used in various domains.
Q: How is Python different from other programming languages?
- A: Python is known for its clear and concise syntax, which emphasizes readability and reduces the cost of program maintenance. It supports both procedural and object-oriented programming.
Python Syntax and Structure:
Q: What is an identifier in Python?
- A: An identifier is a name given to entities in Python, such as variables, functions, classes, etc. It follows certain rules like starting with a letter or underscore.
Q: How does Python use indentation for code structure?
- A: Python uses indentation to define code blocks, replacing curly braces or keywords. Proper indentation is crucial for the code to be syntactically correct.
Q: What are the main data types in Python?
- A: Common data types include int (integer), float (floating-point), str (string), bool (boolean), list, tuple, set, and dict (dictionary).
Python Development Environment:
Q: What is the purpose of a virtual environment in Python?
- A: A virtual environment isolates a Python project, managing dependencies and avoiding conflicts between different projects.
Q: Can you explain the role of the Python Package Index (PyPI)?
- A: PyPI is a repository of Python software packages. It simplifies the process of installing and managing third-party libraries using tools like pip.
Python Variables and Operators:
Q: How do you declare and initialize a variable in Python?
- A: You can declare and initialize a variable like this:
name = "Python"
.
Q: What are the arithmetic operators in Python?
- A: Arithmetic operators include
+
(addition),-
(subtraction),*
(multiplication),/
(division),%
(modulo), and**
(exponentiation).
Python Control Flow:
Q: What is the purpose of the
if
statement in Python?- A: The
if
statement is used for conditional execution. It allows the program to take different paths based on whether a given condition is true or false.
Q: How does the
for
loop work in Python?- A: The
for
loop iterates over a sequence (e.g., a list or string), executing a block of code for each item in the sequence.
Q: Can you explain the role of the
while
loop in Python?- A: The
while
loop repeatedly executes a block of code as long as a specified condition is true.
Functions and Modules:
Q: What is a function in Python?
- A: A function is a reusable block of code that performs a specific task. It can take parameters and may return a value.
Q: How do you import a module in Python?
- A: You can import a module using the
import
keyword. For example:import math
.
Error Handling:
- Q: What is an exception in Python, and how is it handled?
- A: An exception is an error that occurs during the execution of a program. Exception handling in Python involves using
try
,except
, and optionally,finally
blocks.
File Handling:
Q: How do you open and read a file in Python?
- A: You can open and read a file using the
open()
function and methods likeread()
orreadlines()
.
Q: What is the purpose of the
with
statement in file handling?- A: The
with
statement ensures proper handling of resources (like file objects) by automatically closing them when the block is exited.
Object-Oriented Programming (OOP):
Q: What is a class in Python?
- A: A class is a blueprint for creating objects. It defines attributes and methods that the objects created from the class will have.
Q: How does inheritance work in Python?
- A: Inheritance allows a class (subclass) to inherit attributes and methods from another class (superclass), promoting code reusability.
Miscellaneous:
- Q: How can you comment out code in Python?
- A: You can use the
#
symbol for single-line comments, and triple-quotes ('''
or"""
) for multi-line comments.
Summary
The Python programming language is known for its simplicity and readability, making it a popular choice for beginners and experienced developers alike. It is a high-level, interpreted language that emphasizes code readability and simplicity, which allows programmers to express concepts in fewer lines of code compared to other languages.
Python supports multiple programming paradigms, including procedural, object-oriented, and functional programming. It has a large standard library that provides support for many common tasks, such as file I/O, networking, and data manipulation.
One of the key features of Python is its dynamic typing system, which means that variables do not need to be explicitly declared. This makes Python code more flexible and easier to read, but it can also lead to potential runtime errors if not used carefully.
Python's syntax uses indentation to define code blocks, which enforces a clean and consistent coding style. This can be a benefit for readability, but it can also be a source of frustration for developers coming from other languages that use braces or keywords to define blocks.
Overall, Python is a versatile and powerful language that is widely used in a variety of applications, including web development, data analysis, artificial intelligence, and scientific computing. Its simplicity and readability make it an excellent choice for both beginners and experienced developers