Control Structures
In Python, control structures are used to alter the flow of execution in a program. They include conditional statements (if
, elif
, else
), loops (for
, while
), and control flow modifiers (break
, continue
, pass
, return
). These structures allow you to make decisions, repeat blocks of code, and handle exceptional cases in your code
Table of contents:
1.Introduction to Control Structures
- What are control structures?
- Why are control structures important in programming?
- Overview of control structures in Python.
2.Conditional Statements
if
statementelif
statementelse
statement- Examples and use cases
3.Loops
for
loopwhile
loop- Nested loops
- Loop control statements (
break
,continue
) - Examples and use cases
4.Control Flow Modifiers
pass
statementreturn
statement- Examples and use cases
5.Error Handling with Control Structures
try
,except
,else
,finally
blocks- Handling exceptions in Python
- Examples and use cases
6.Best Practices for Using Control Structures
- Guidelines for writing clean and readable code
- Avoiding common pitfalls
- Tips for optimizing control structures
7.Advanced Control Structures
- List comprehensions
- Generator expressions
- Recursive functions
- Examples and use cases
8.Case Studies and Examples
- Real-world examples showcasing the use of control structures
- Problem-solving with control structures
- Code snippets and explanations
9.Conclusion
- Recap of key concepts
- Final thoughts on using control structures effectively in Python
10.Appendix
- Additional resources
- Glossary of key terms
- Exercises and solutions
Types of Control Structures:
- Conditional Statements
- Loops
- Control Flow Modifiers
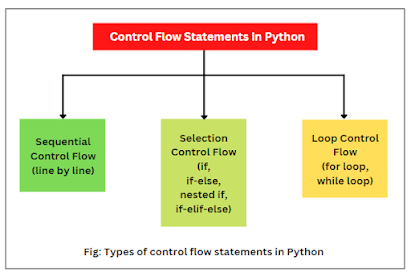
if
statement: Executes a block of code only if a specified condition is true. Ex:
x = 10
if x > 5: print("x is greater than 5")
elif
statement: Allows you to check multiple conditions after an initialif
statement.- Ex:
else
statement:- Executes a block of code if the preceding
if
orelif
conditions are not met. - Ex:
if x > 5:
print("x is greater than 5")
elif x < 5: print("x is less than 5")
if x > 5:print("x is greater than 5")else:print("x is 5 or less")
Loops:
for
loop:
Iterates over a sequence (e.g., list, tuple, string) or other iterable objects.
- Ex:
fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
while
loop:- Repeats a block of code as long as a specified condition is true.
- Ex:
i = 0
while i < 5:
print(i)
i += 1
Control Flow Modifiers:
break
statement:
Terminates the loop it is in and transfers control to the next statement outside the loop.
Ex:
for i in range(5):
print(i)
if i == 2:
break
continue
statement:
Skips the rest of the code inside a loop for the current iteration and goes to the next iteration.
Ex:
for i in range(5):if i == 2:
continue
print(i)
pass
statement: x = 10if x > 5:pass
return
statement: def add(a, b):return a + bresult = add(3, 5)print(result)
Uses of control structures
Conditional Execution:
Control structures like
if
,elif
, andelse
allow you to execute certain blocks of code based on specified conditions. This is useful for implementing decision-making logic in your programs.Looping:
Control structures such as
for
andwhile
loops allow you to repeat a block of code multiple times. This is useful for iterating over sequences, processing data, and implementing repetitive tasks.Control Flow Modification:
Statements like
break
,continue
, andpass
allow you to modify the flow of control in your program. For example,break
can be used to exit a loop prematurely,continue
can be used to skip the rest of the loop and start the next iteration, andpass
can be used as a placeholder when no action is needed.Error Handling:
Control structures can be used to handle exceptions and errors in your code. For example,
try
andexcept
blocks can be used to catch and handle exceptions, preventing your program from crashing.Function and Module Execution:
Control structures are used to define functions and modules in Python. Functions allow you to encapsulate code for reuse, while modules allow you to organize related code into separate files.
Data Filtering and Transformation:
Control structures are often used to filter and transform data. For example, you can use a loop to iterate over a list of numbers and filter out the even numbers or transform them into a new list.
- User Input Validation:
Control structures can be used to validate user input. For example, you can use a loop to repeatedly ask the user for input until they provide a valid response.
State Management:
Control structures can be used to manage the state of a program. For example, you can use a series of
if
statements to check different conditions and update the program state accordingly.Concurrency and Parallelism:
Control structures can be used to manage concurrent and parallel execution in Python. For example, you can use the
threading
ormultiprocessing
modules to create and manage threads or processes.Event Handling:
Control structures can be used to handle events in graphical user interfaces (GUIs) or other event-driven programming paradigms. For example, you can use
if
statements to check for specific events and trigger corresponding actions.Algorithm Implementation:
Control structures are used extensively in implementing algorithms and data structures. For example, you can use loops and conditional statements to implement sorting algorithms, searching algorithms, and more.
Code Organization and Readability:
Control structures can improve the organization and readability of your code. For example, using meaningful variable names and properly indenting your code can make it easier to understand and maintain.
- Key points
Conditional Statements (
if
,elif
,else
):- Execute different blocks of code based on conditions.
elif
allows checking multiple conditions.else
is executed if no conditions are true.
Loops (
for
,while
):for
iterates over sequences or iterable objects.while
repeats a block of code while a condition is true.break
exits the loop.continue
skips the rest of the code in the current iteration.
Control Flow Modifiers (
pass
,return
):pass
is a placeholder when no action is needed.return
exits a function and optionally returns a value.
Uses of Control Structures:
- Conditionally execute code.
- Repeat code using loops.
- Modify flow with
break
,continue
. - Handle errors and exceptions.
- Validate input, filter data, manage state.
- Implement algorithms, organize code, improve readability.
Real Time Problems Statements On Control Structures
Conditional Statements:
- Write a program that takes a number as input and prints whether it is positive, negative, or zero.
- Write a program that takes a year as input and determines whether it is a leap year or not.
- Write a program that takes a string as input and determines whether it is a palindrome or not.
Loops:
- Write a program that prints the multiplication table of a given number.
- Write a program that calculates the factorial of a given number.
- Write a program that generates the Fibonacci sequence up to a certain number of terms.
Control Flow Modifiers:
- Write a program that finds the sum of all numbers between 1 and 100 that are divisible by 3.
- Write a program that finds the largest element in a list.
- Write a program that takes a list of numbers as input and calculates the average.
Combining Control Structures:
- Write a program that takes a list of numbers as input and prints all the even numbers.
- Write a program that takes a list of strings as input and prints the longest string.
- Write a program that takes a number as input and prints all the prime numbers up to that number.
Error Handling:
- Write a program that takes two numbers as input and divides them, handling the
ZeroDivisionError
exception if the second number is zero. - Write a program that takes a list of numbers as input and calculates the sum, handling the
ValueError
exception if any of the inputs are not numbers.
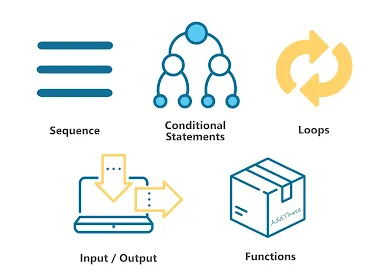
Faq's
How do you use the for
loop in Python?
- The
for
loop in Python is used to iterate over a sequence or iterable object. It iterates over each item in the sequence and executes the block of code inside the loop for each item. For example: - for item in iterable: # Code to execute for each item
How does the while
loop work in Python?
- The
while
loop in Python repeats a block of code as long as a specified condition is true. It evaluates the condition before each iteration. - For example:
- while condition: # Code to execute as long as the condition is true
What is the difference between
for
andwhile
loops in Python?- The
for
loop is used for iterating over a sequence, while thewhile
loop is used for repeated execution based on a condition. Thefor
loop iterates over a fixed sequence, whereas thewhile
loop continues until a condition becomes false.
How do you exit a loop in Python?
- You can exit a loop in Python using the
break
statement. When thebreak
statement is encountered inside a loop, the loop is immediately terminated, and the program execution continues with the next statement after the loop.
How do you skip the rest of the code in a loop and continue to the next iteration in Python?
- You can skip the rest of the code in a loop for the current iteration and continue to the next iteration using the
continue
statement. When thecontinue
statement is encountered inside a loop, the rest of the code in the loop for the current iteration is skipped, and the loop continues with the next iteration.
What is the purpose of the
pass
statement in Python?- The
pass
statement in Python is used as a placeholder when no action is required. It is a null operation; nothing happens when it is executed. Thepass
statement is often used as a placeholder in empty code blocks, such as inif
statements, loops, or function definitions.
When should you use the
pass
statement in Python?- You should use the
pass
statement in Python when you need a syntactically correct empty code block but do not want to perform any action. It is often used as a placeholder that allows you to define a function, class, or control structure without implementing its functionality immediately.
How do you return a value from a function in Python?
- You can return a value from a function in Python using the
return
statement. Thereturn
statement is followed by the value that you want to return. When thereturn
statement is executed, the function terminates, and the value is returned to the caller. - For example:
- def add(a, b): return a + b result = add(3, 5) print(result) # Output: 8
What is the purpose of the
return
statement in Python?- The
return
statement in Python is used to exit a function and return a value to the caller. It allows you to pass back a result or data from the function to the code that called the function.
Can you use multiple
if
statements in a row withoutelif
orelse
in Python?- Yes, you can use multiple
if
statements in a row withoutelif
orelse
in Python. Eachif
statement is evaluated independently, so allif
statements that evaluate to true will execute their corresponding blocks of code. However, usingelif
andelse
can often make the code more readable and efficient.
How do you handle exceptions in Python using control structures?
- You can handle exceptions in Python using
try
,except
,else
, andfinally
blocks. Thetry
block is used to wrap the code that may raise an exception. Theexcept
block is used to handle specific exceptions that occur within thetry
block. Theelse
block is executed if no exceptions are raised in thetry
block. Thefinally
block is always executed, regardless of whether an exception occurs or not.
What are some common uses of control structures in Python?
- Some common uses of control structures in Python include:
- Implementing conditional logic to execute different blocks of code based on conditions.
- Iterating over sequences or iterable objects to process data.
- Modifying the flow of control in a program using
break
,continue
, andpass
. - Handling errors and exceptions.
- Validating user input.
- Filtering and transforming data.
- Managing program state.
- Implementing algorithms and data structures.
How do you validate user input using control structures in Python?
- You can validate user input using control structures in Python by using
if
statements to check the input against certain criteria. For example, you can check if a user-entered value is a valid number or if it falls within a certain range. If the input is not valid, you can prompt the user to enter a valid value.
Can you nest control structures in Python?
- Yes, you can nest control structures in Python. For example, you can nest an
if
statement inside anotherif
statement, or you can nest a loop inside another loop. Nesting control structures allows you to create complex logic and handle various scenarios in your code.
How do you organize complex logic using control structures in Python?
- You can organize complex logic using control structures in Python by breaking it down into smaller, more manageable parts. You can use functions to encapsulate and reuse code, and you can use control structures such as
if
statements, loops, and control flow modifiers to control the flow of execution based on different conditions.
What is the purpose of the
continue
statement in Python?- The
continue
statement in Python is used to skip the rest of the code inside a loop for the current iteration and continue with the next iteration. It is often used to skip certain iterations of a loop based on a condition.
How do you use the
break
statement to exit a loop in Python?- You can use the
break
statement in Python to exit a loop prematurely. When thebreak
statement is encountered inside a loop, the loop is immediately terminated, and the program execution continues with the next statement after the loop.
How do you create an infinite loop in Python?
- You can create an infinite loop in Python by using a
while
loop with a condition that always evaluates toTrue
.
- For example:
- while True: # Code that runs indefinitely
How do you iterate over a dictionary using control structures in Python?
for
loop. By default, the for
loop iterates over the keys of the dictionary. - For example:
my_dict = {'a': 1, 'b': 2, 'c': 3}for key in my_dict:print(key, my_dict[key])
What is the role of control structures in algorithm design in Python?
Conditional statements, such as if
, elif
, and else
, allow developers to execute different blocks of code based on specified conditions. This is useful for implementing decision-making logic in programs.
Loops, such as for
and while
, enable developers to repeat a block of code multiple times. for
loops iterate over a sequence or iterable object, while while
loops repeat as long as a specified condition is true. Control flow modifiers like break
and continue
allow developers to modify the flow of control within loops. break
exits the loop, while continue
skips the rest of the code in the current iteration and continues to the next iteration.
Control structures are used in various ways in Python programming. They are used to handle errors and exceptions, validate user input, filter and transform data, manage program state, and implement algorithms and data structures. Control structures also play a role in organizing code and improving readability.
Understanding and using control structures effectively is essential for writing clear, efficient, and maintainable Python code. Developers should be familiar with the different types of control structures available in Python and how they can be used to solve various programming problems.
๐๐๐๐๐